Spaces:
Running
Running
Update chatbot.py
Browse files- chatbot.py +308 -134
chatbot.py
CHANGED
@@ -1,12 +1,10 @@
|
|
1 |
import os
|
2 |
import time
|
3 |
-
import copy
|
4 |
import requests
|
5 |
import random
|
6 |
from threading import Thread
|
7 |
from typing import List, Dict, Union
|
8 |
import subprocess
|
9 |
-
# Install flash attention, skipping CUDA build if necessary
|
10 |
subprocess.run(
|
11 |
"pip install flash-attn --no-build-isolation",
|
12 |
env={"FLASH_ATTENTION_SKIP_CUDA_BUILD": "TRUE"},
|
@@ -15,7 +13,6 @@ subprocess.run(
|
|
15 |
import torch
|
16 |
import gradio as gr
|
17 |
from bs4 import BeautifulSoup
|
18 |
-
import datasets
|
19 |
from transformers import LlavaProcessor, LlavaForConditionalGeneration, TextIteratorStreamer
|
20 |
from huggingface_hub import InferenceClient
|
21 |
from PIL import Image
|
@@ -23,17 +20,24 @@ import spaces
|
|
23 |
from functools import lru_cache
|
24 |
import cv2
|
25 |
import re
|
26 |
-
import io
|
|
|
|
|
|
|
27 |
|
28 |
# You can also use models that are commented below
|
29 |
# model_id = "llava-hf/llava-interleave-qwen-0.5b-hf"
|
30 |
model_id = "llava-hf/llava-interleave-qwen-7b-hf"
|
31 |
# model_id = "llava-hf/llava-interleave-qwen-7b-dpo-hf"
|
32 |
processor = LlavaProcessor.from_pretrained(model_id)
|
33 |
-
model = LlavaForConditionalGeneration.from_pretrained(model_id,
|
34 |
model.to("cuda")
|
35 |
# Credit to merve for code of llava interleave qwen
|
36 |
|
|
|
|
|
|
|
|
|
37 |
def sample_frames(video_file) :
|
38 |
try:
|
39 |
video = cv2.VideoCapture(video_file)
|
@@ -60,61 +64,59 @@ examples_path = os.path.dirname(__file__)
|
|
60 |
EXAMPLES = [
|
61 |
[
|
62 |
{
|
63 |
-
"text": "
|
64 |
}
|
65 |
],
|
66 |
[
|
67 |
{
|
68 |
-
"text": "
|
69 |
}
|
70 |
],
|
71 |
[
|
72 |
{
|
73 |
-
"text": "What
|
74 |
-
"files": [f"{examples_path}/example_video/accident.gif"],
|
75 |
}
|
76 |
],
|
77 |
[
|
78 |
{
|
79 |
-
"text": "
|
80 |
-
"files": [f"{examples_path}/example_video/spiderman.gif"],
|
81 |
}
|
82 |
],
|
83 |
[
|
84 |
{
|
85 |
-
"text": "
|
86 |
-
"files": [f"{examples_path}/example_images/paper_with_text.png"],
|
87 |
}
|
88 |
],
|
89 |
[
|
90 |
{
|
91 |
-
"text": "
|
92 |
-
"files": [f"{examples_path}/example_images/elon_smoking.jpg",
|
93 |
-
f"{examples_path}/example_images/steve_jobs.jpg", ]
|
94 |
}
|
95 |
],
|
96 |
[
|
97 |
{
|
98 |
-
"text": "
|
|
|
99 |
}
|
100 |
],
|
101 |
[
|
102 |
{
|
103 |
-
"text": "
|
|
|
104 |
}
|
105 |
],
|
106 |
[
|
107 |
{
|
108 |
-
"text": "
|
109 |
-
"files": [f"{examples_path}/example_images/
|
110 |
}
|
111 |
],
|
112 |
[
|
113 |
{
|
114 |
-
"text": "
|
115 |
-
"files": [f"{examples_path}/example_images/
|
|
|
116 |
}
|
117 |
-
]
|
118 |
]
|
119 |
|
120 |
# Set bot avatar image
|
@@ -131,139 +133,90 @@ def extract_text_from_webpage(html_content):
|
|
131 |
return visible_text
|
132 |
|
133 |
# Perform a Google search and return the results
|
134 |
-
def search(
|
135 |
-
|
136 |
start = 0
|
137 |
all_results = []
|
138 |
-
|
139 |
-
|
140 |
-
|
141 |
-
with requests.Session() as session:
|
142 |
-
resp = session.get(
|
143 |
url="https://www.google.com/search",
|
144 |
-
headers={"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/111.0"},
|
145 |
-
|
146 |
-
|
147 |
-
|
148 |
-
"udm": 14,
|
149 |
-
},
|
150 |
-
timeout=timeout,
|
151 |
-
verify=ssl_verify,
|
152 |
)
|
153 |
resp.raise_for_status()
|
154 |
soup = BeautifulSoup(resp.text, "html.parser")
|
155 |
result_block = soup.find_all("div", attrs={"class": "g"})
|
156 |
for result in result_block:
|
157 |
link = result.find("a", href=True)
|
158 |
-
|
159 |
-
|
160 |
-
|
161 |
-
|
162 |
-
|
163 |
-
|
164 |
-
|
165 |
-
|
166 |
-
|
167 |
-
|
168 |
-
except requests.exceptions.RequestException as e:
|
169 |
-
print(f"Error fetching or processing {link}: {e}")
|
170 |
-
all_results.append({"link": link, "text": None})
|
171 |
-
else:
|
172 |
-
all_results.append({"link": None, "text": None})
|
173 |
return all_results
|
174 |
|
175 |
-
# Format the prompt for the language model
|
176 |
-
def format_prompt(user_prompt, chat_history):
|
177 |
-
prompt = "<s>"
|
178 |
-
for item in chat_history:
|
179 |
-
# Check if the item is a tuple (text response)
|
180 |
-
if isinstance(item, tuple):
|
181 |
-
prompt += f"[INST] {item[0]} [/INST]" # User prompt
|
182 |
-
prompt += f" {item[1]}</s> " # Bot response
|
183 |
-
# Otherwise, assume it's related to an image - you might need to adjust this logic
|
184 |
-
else:
|
185 |
-
# Handle image representation in the prompt, e.g., add a placeholder
|
186 |
-
prompt += f" [Image] "
|
187 |
-
prompt += f"[INST] {user_prompt} [/INST]"
|
188 |
-
return prompt
|
189 |
-
|
190 |
-
|
191 |
-
client_mixtral = InferenceClient("NousResearch/Nous-Hermes-2-Mixtral-8x7B-DPO")
|
192 |
-
client_mistral = InferenceClient("HuggingFaceH4/zephyr-7b-beta")
|
193 |
-
generate_kwargs = dict( max_new_tokens=4000, do_sample=True, stream=True, details=True, return_full_text=False )
|
194 |
-
|
195 |
-
system_llava = "<|im_start|>system\nYou are OpenGPT 4o, an exceptionally capable and versatile AI assistant made by KingNish. Your task is to fulfill users query in best possible way. You are provided with image, videos and 3d structures as input with question your task is to give best possible detailed results to user according to their query. Reply the question asked by user properly and best possible way.<|im_end|>"
|
196 |
-
|
197 |
-
@spaces.GPU(duration=60, queue=False)
|
198 |
-
def model_inference( user_prompt, chat_history, web_search):
|
199 |
-
if not user_prompt["files"]:
|
200 |
-
if web_search is True:
|
201 |
-
|
202 |
-
gr.Info("Searching Web")
|
203 |
-
|
204 |
-
web_results = search(user_prompt["text"])
|
205 |
-
|
206 |
-
gr.Info("Extracting relevant Info")
|
207 |
-
|
208 |
-
web2 = ' '.join([f"Link: {res['link']}\nText: {res['text']}\n\n" for res in web_results])
|
209 |
-
|
210 |
-
messages = f"<|im_start|>system\nYou are OpenGPT 4o, an exceptionally capable and versatile AI assistant meticulously crafted by KingNish. You are provided with WEB results from which you can find informations to answer users query in Structured and More better way. You do not say Unnecesarry things Only say thing which is important and relevant. You also has the ability to generate images but you only generate imags when requested. BY Utilizing the following link structure, : 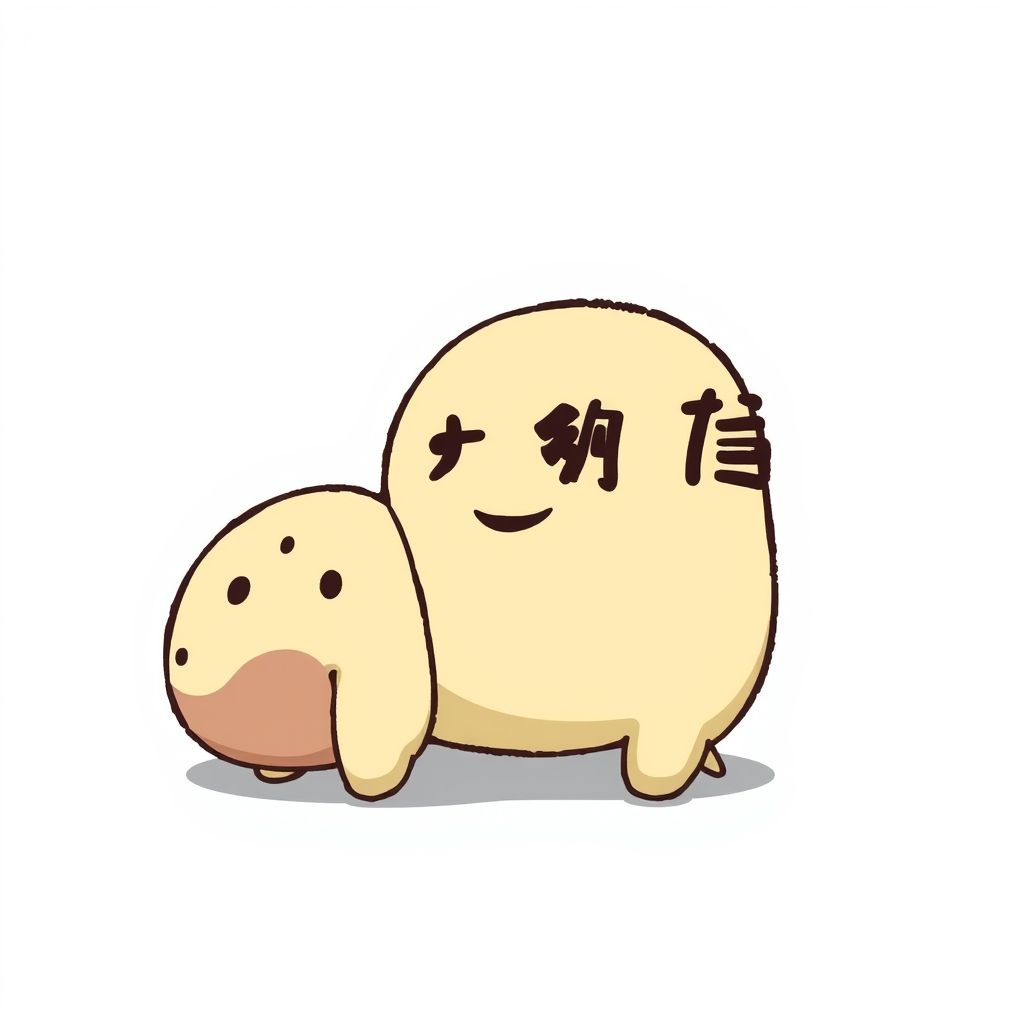 For image generation, You replace info inside bracets with specific details according to their requiremnts to create relevant visuals. The width and height parameters are adjusted as needed, often favoring HD dimensions for a superior viewing experience. Note: 1. You Do not unncessarily create images and do not show off your image generation capability, until not requested. 2. You always Give image link in format  Make sure to not generate image until requested 3. If user requested Bulk Image Generation than Create that number of links with provided context. 4. Always learn from previous conversation. 5. Always try to connect conversation with history. 6. Do not make conversation too long. 7. Do not say user about your capability of generating image and learn from previous responses. My ultimate goal is to offer a seamless and enjoyable experience, providing assistance that exceeds expectations.You remain a reliable and trusted companion to the User. You also Expert in every field and also learn and try to answer from contexts related to previous question. Make sure to not generate image until requested. and Try your best to give best response possible.<|im_end|>"
|
211 |
|
212 |
-
|
213 |
-
|
214 |
-
|
215 |
|
216 |
-
|
217 |
-
|
218 |
-
|
219 |
-
# Construct the output from the stream of tokens
|
220 |
-
for response in stream:
|
221 |
-
if not response.token.text == "<|im_end|>":
|
222 |
-
output += response.token.text
|
223 |
-
yield output
|
224 |
-
else:
|
225 |
-
messages = f"<|im_start|>system\nYou are OpenGPT 4o, an exceptionally capable and versatile AI assistant meticulously crafted by KingNish. You do not say Unnecesarry things Only say thing which is important and relevant. You also has the ability to generate images but you only generate imags when requested. BY Utilizing the following link structure, : 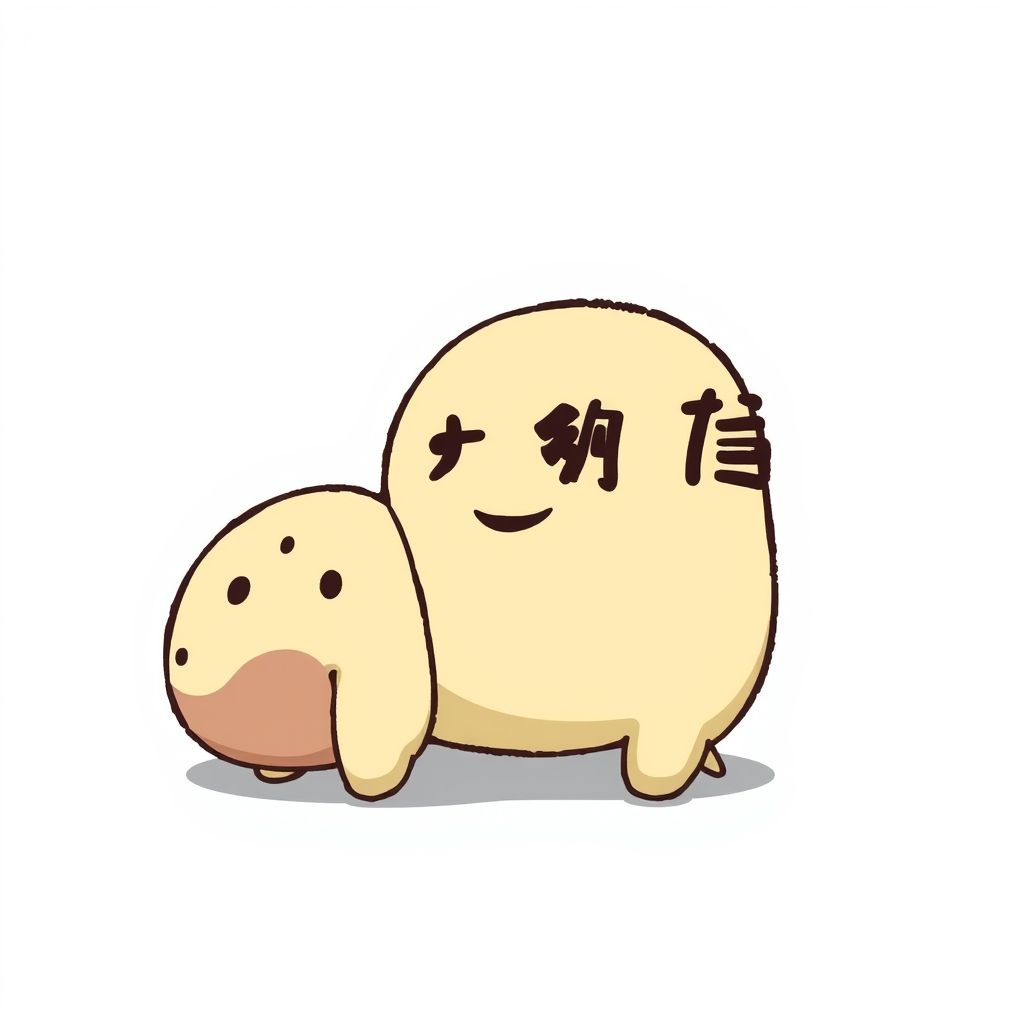 For image generation, You replace info inside bracets with specific details according to their requiremnts to create relevant visuals. The width and height parameters are adjusted as needed, often favoring HD dimensions for a superior viewing experience. Note: 1. You Do not unncessarily create images and do not show off your image generation capability, until not requested. 2. You always Give image link in format  3. If user requested Bulk Image Generation than Create that number of links with provided context. 4. Always learn from previous conversation. 5. Always try to connect conversation with history. 6. Do not make conversation too long. 7. Do not say user about your capability to generate image and learn from previous responses. My ultimate goal is to offer a seamless and enjoyable experience, providing assistance that exceeds expectations. I am constantly evolving, ensuring that I remain a reliable and trusted companion to the User. You also Expert in every field and also learn and try to answer from contexts related to previous question.<|im_end|>"
|
226 |
-
|
227 |
-
for msg in chat_history:
|
228 |
-
messages += f"\n<|im_start|>user\n{str(msg[0])}<|im_end|>"
|
229 |
-
messages += f"\n<|im_start|>assistant\n{str(msg[1])}<|im_end|>"
|
230 |
-
|
231 |
-
messages+=f"\n<|im_start|>user\n{user_prompt}<|im_end|>\n<|im_start|>assistant\n"
|
232 |
|
233 |
-
|
234 |
-
|
235 |
-
|
236 |
-
for response in stream:
|
237 |
-
if not response.token.text == "<|im_end|>":
|
238 |
-
output += response.token.text
|
239 |
-
yield output
|
240 |
else:
|
241 |
-
|
|
|
|
|
242 |
|
243 |
-
|
244 |
-
|
245 |
-
ext_buffer =f"'user\ntext': '{txt}', 'files': '{img}' assistant"
|
246 |
|
247 |
-
|
248 |
-
|
249 |
-
|
250 |
|
251 |
-
|
252 |
-
|
253 |
-
|
254 |
-
|
255 |
-
|
256 |
|
257 |
-
|
258 |
-
|
259 |
-
|
|
|
|
|
|
|
260 |
|
261 |
-
|
262 |
|
263 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
264 |
streamer = TextIteratorStreamer(processor, skip_prompt=True, **{"skip_special_tokens": True})
|
265 |
generation_kwargs = dict(inputs, streamer=streamer, max_new_tokens=2048)
|
266 |
-
generated_text = ""
|
267 |
|
268 |
thread = Thread(target=model.generate, kwargs=generation_kwargs)
|
269 |
thread.start()
|
@@ -273,12 +226,233 @@ def model_inference( user_prompt, chat_history, web_search):
|
|
273 |
buffer += new_text
|
274 |
yield buffer
|
275 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
276 |
# Create a chatbot interface
|
277 |
chatbot = gr.Chatbot(
|
278 |
label="OpenGPT-4o",
|
279 |
avatar_images=[None, BOT_AVATAR],
|
280 |
show_copy_button=True,
|
281 |
likeable=True,
|
282 |
-
layout="panel"
|
|
|
283 |
)
|
284 |
output = gr.Textbox(label="Prompt")
|
|
|
1 |
import os
|
2 |
import time
|
|
|
3 |
import requests
|
4 |
import random
|
5 |
from threading import Thread
|
6 |
from typing import List, Dict, Union
|
7 |
import subprocess
|
|
|
8 |
subprocess.run(
|
9 |
"pip install flash-attn --no-build-isolation",
|
10 |
env={"FLASH_ATTENTION_SKIP_CUDA_BUILD": "TRUE"},
|
|
|
13 |
import torch
|
14 |
import gradio as gr
|
15 |
from bs4 import BeautifulSoup
|
|
|
16 |
from transformers import LlavaProcessor, LlavaForConditionalGeneration, TextIteratorStreamer
|
17 |
from huggingface_hub import InferenceClient
|
18 |
from PIL import Image
|
|
|
20 |
from functools import lru_cache
|
21 |
import cv2
|
22 |
import re
|
23 |
+
import io
|
24 |
+
import json
|
25 |
+
from gradio_client import Client, file
|
26 |
+
from groq import Groq
|
27 |
|
28 |
# You can also use models that are commented below
|
29 |
# model_id = "llava-hf/llava-interleave-qwen-0.5b-hf"
|
30 |
model_id = "llava-hf/llava-interleave-qwen-7b-hf"
|
31 |
# model_id = "llava-hf/llava-interleave-qwen-7b-dpo-hf"
|
32 |
processor = LlavaProcessor.from_pretrained(model_id)
|
33 |
+
model = LlavaForConditionalGeneration.from_pretrained(model_id,torch_dtype=torch.float16, use_flash_attention_2=True, low_cpu_mem_usage=True)
|
34 |
model.to("cuda")
|
35 |
# Credit to merve for code of llava interleave qwen
|
36 |
|
37 |
+
GROQ_API_KEY = os.environ.get("GROQ_API_KEY", None)
|
38 |
+
|
39 |
+
client_groq = Groq(api_key=GROQ_API_KEY)
|
40 |
+
|
41 |
def sample_frames(video_file) :
|
42 |
try:
|
43 |
video = cv2.VideoCapture(video_file)
|
|
|
64 |
EXAMPLES = [
|
65 |
[
|
66 |
{
|
67 |
+
"text": "What is Friction? Explain in Detail.",
|
68 |
}
|
69 |
],
|
70 |
[
|
71 |
{
|
72 |
+
"text": "Write me a Python function to generate unique passwords.",
|
73 |
}
|
74 |
],
|
75 |
[
|
76 |
{
|
77 |
+
"text": "What's the latest price of Bitcoin?",
|
|
|
78 |
}
|
79 |
],
|
80 |
[
|
81 |
{
|
82 |
+
"text": "Search and give me list of spaces trending on HuggingFace.",
|
|
|
83 |
}
|
84 |
],
|
85 |
[
|
86 |
{
|
87 |
+
"text": "Create image of a Beauiful white colour supercar.",
|
|
|
88 |
}
|
89 |
],
|
90 |
[
|
91 |
{
|
92 |
+
"text": "Create image of cute happy robot.",
|
|
|
|
|
93 |
}
|
94 |
],
|
95 |
[
|
96 |
{
|
97 |
+
"text": "What unusual happens in this video.",
|
98 |
+
"files": [f"{examples_path}/example_video/accident.gif"],
|
99 |
}
|
100 |
],
|
101 |
[
|
102 |
{
|
103 |
+
"text": "What's name of superhero in this clip",
|
104 |
+
"files": [f"{examples_path}/example_video/spiderman.gif"],
|
105 |
}
|
106 |
],
|
107 |
[
|
108 |
{
|
109 |
+
"text": "What's written on this paper",
|
110 |
+
"files": [f"{examples_path}/example_images/paper_with_text.png"],
|
111 |
}
|
112 |
],
|
113 |
[
|
114 |
{
|
115 |
+
"text": "Who are they? Tell me about both of them",
|
116 |
+
"files": [f"{examples_path}/example_images/elon_smoking.jpg",
|
117 |
+
f"{examples_path}/example_images/steve_jobs.jpg", ]
|
118 |
}
|
119 |
+
]
|
120 |
]
|
121 |
|
122 |
# Set bot avatar image
|
|
|
133 |
return visible_text
|
134 |
|
135 |
# Perform a Google search and return the results
|
136 |
+
def search(query):
|
137 |
+
term = query
|
138 |
start = 0
|
139 |
all_results = []
|
140 |
+
max_chars_per_page = 6000
|
141 |
+
with requests.Session() as session:
|
142 |
+
resp = session.get(
|
|
|
|
|
143 |
url="https://www.google.com/search",
|
144 |
+
headers={"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/111.0"},
|
145 |
+
params={"q": term, "num": 4, "udm": 14},
|
146 |
+
timeout=5,
|
147 |
+
verify=None,
|
|
|
|
|
|
|
|
|
148 |
)
|
149 |
resp.raise_for_status()
|
150 |
soup = BeautifulSoup(resp.text, "html.parser")
|
151 |
result_block = soup.find_all("div", attrs={"class": "g"})
|
152 |
for result in result_block:
|
153 |
link = result.find("a", href=True)
|
154 |
+
link = link["href"]
|
155 |
+
try:
|
156 |
+
webpage = session.get(link, headers={"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/111.0"}, timeout=5, verify=False)
|
157 |
+
webpage.raise_for_status()
|
158 |
+
visible_text = extract_text_from_webpage(webpage.text)
|
159 |
+
if len(visible_text) > max_chars_per_page:
|
160 |
+
visible_text = visible_text[:max_chars_per_page]
|
161 |
+
all_results.append({"link": link, "text": visible_text})
|
162 |
+
except requests.exceptions.RequestException:
|
163 |
+
all_results.append({"link": link, "text": None})
|
|
|
|
|
|
|
|
|
|
|
164 |
return all_results
|
165 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
166 |
|
167 |
+
def image_gen(prompt):
|
168 |
+
client = Client("KingNish/Image-Gen-Pro")
|
169 |
+
return client.predict("Image Generation",None, prompt, api_name="/image_gen_pro")
|
170 |
|
171 |
+
def video_gen(prompt):
|
172 |
+
client = Client("KingNish/Instant-Video")
|
173 |
+
return client.predict(prompt, api_name="/instant_video")
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
174 |
|
175 |
+
def llava(user_prompt, chat_history):
|
176 |
+
if user_prompt["files"]:
|
177 |
+
image = user_prompt["files"][0]
|
|
|
|
|
|
|
|
|
178 |
else:
|
179 |
+
for hist in chat_history:
|
180 |
+
if type(hist[0])==tuple:
|
181 |
+
image = hist[0][0]
|
182 |
|
183 |
+
txt = user_prompt["text"]
|
184 |
+
img = user_prompt["files"]
|
|
|
185 |
|
186 |
+
video_extensions = ("avi", "mp4", "mov", "mkv", "flv", "wmv", "mjpeg", "wav", "gif", "webm", "m4v", "3gp")
|
187 |
+
image_extensions = Image.registered_extensions()
|
188 |
+
image_extensions = tuple([ex for ex, f in image_extensions.items()])
|
189 |
|
190 |
+
if image.endswith(video_extensions):
|
191 |
+
image = sample_frames(image)
|
192 |
+
gr.Info("Analyzing Video")
|
193 |
+
image_tokens = "<image>" * int(len(image))
|
194 |
+
prompt = f"<|im_start|>user {image_tokens}\n{user_prompt}<|im_end|><|im_start|>assistant"
|
195 |
|
196 |
+
elif image.endswith(image_extensions):
|
197 |
+
image = Image.open(image).convert("RGB")
|
198 |
+
gr.Info("Analyzing image")
|
199 |
+
prompt = f"<|im_start|>user <image>\n{user_prompt}<|im_end|><|im_start|>assistant"
|
200 |
+
|
201 |
+
system_llava = "<|im_start|>system\nYou are OpenGPT 4o, an exceptionally capable and versatile AI assistant made by KingNish. Your task is to fulfill users query in best possible way. You are provided with image, videos and 3d structures as input with question your task is to give best possible detailed results to user according to their query. Reply the question asked by user properly and best possible way.<|im_end|>"
|
202 |
|
203 |
+
final_prompt = f"{system_llava}\n{prompt}"
|
204 |
|
205 |
+
inputs = processor(final_prompt, image, return_tensors="pt").to("cuda", torch.float16)
|
206 |
+
|
207 |
+
return inputs
|
208 |
+
|
209 |
+
# Initialize inference clients for different models
|
210 |
+
client_gemma = InferenceClient("mistralai/Mistral-7B-Instruct-v0.3")
|
211 |
+
client_mixtral = InferenceClient("NousResearch/Nous-Hermes-2-Mixtral-8x7B-DPO")
|
212 |
+
client_llama = InferenceClient("meta-llama/Meta-Llama-3-8B-Instruct")
|
213 |
+
|
214 |
+
@spaces.GPU(duration=60, queue=False)
|
215 |
+
def model_inference( user_prompt, chat_history):
|
216 |
+
if user_prompt["files"]:
|
217 |
+
inputs = llava(user_prompt, chat_history)
|
218 |
streamer = TextIteratorStreamer(processor, skip_prompt=True, **{"skip_special_tokens": True})
|
219 |
generation_kwargs = dict(inputs, streamer=streamer, max_new_tokens=2048)
|
|
|
220 |
|
221 |
thread = Thread(target=model.generate, kwargs=generation_kwargs)
|
222 |
thread.start()
|
|
|
226 |
buffer += new_text
|
227 |
yield buffer
|
228 |
|
229 |
+
else:
|
230 |
+
func_caller = []
|
231 |
+
message = user_prompt
|
232 |
+
|
233 |
+
functions_metadata = [
|
234 |
+
{"type": "function", "function": {"name": "web_search", "description": "Search query on google and find latest information, info about any person, object, place thing, everything that available on google.", "parameters": {"type": "object", "properties": {"query": {"type": "string", "description": "web search query"}}, "required": ["query"]}}},
|
235 |
+
{"type": "function", "function": {"name": "general_query", "description": "Reply general query of USER, with LLM like you. But it does not answer tough questions and latest info's.", "parameters": {"type": "object", "properties": {"prompt": {"type": "string", "description": "A detailed prompt"}}, "required": ["prompt"]}}},
|
236 |
+
{"type": "function", "function": {"name": "hard_query", "description": "Reply tough query of USER, using powerful LLM. But it does not answer latest info's.", "parameters": {"type": "object", "properties": {"prompt": {"type": "string", "description": "A detailed prompt"}}, "required": ["prompt"]}}},
|
237 |
+
{"type": "function", "function": {"name": "image_generation", "description": "Generate image for user", "parameters": {"type": "object", "properties": {"query": {"type": "string", "description": "image generation prompt"}}, "required": ["query"]}}},
|
238 |
+
{"type": "function", "function": {"name": "video_generation", "description": "Generate video for user", "parameters": {"type": "object", "properties": {"query": {"type": "string", "description": "video generation prompt"}}, "required": ["query"]}}},
|
239 |
+
{"type": "function", "function": {"name": "image_qna", "description": "Answer question asked by user related to image", "parameters": {"type": "object", "properties": {"query": {"type": "string", "description": "Question by user"}}, "required": ["query"]}}},
|
240 |
+
]
|
241 |
+
|
242 |
+
for msg in chat_history:
|
243 |
+
func_caller.append({"role": "user", "content": f"{str(msg[0])}"})
|
244 |
+
func_caller.append({"role": "assistant", "content": f"{str(msg[1])}"})
|
245 |
+
|
246 |
+
message_text = message["text"]
|
247 |
+
func_caller.append({"role": "user", "content": f'[SYSTEM]You are a helpful assistant. You have access to the following functions: \n {str(functions_metadata)}\n\nTo use these functions respond with:\n<functioncall> {{ "name": "function_name", "arguments": {{ "arg_1": "value_1", "arg_1": "value_1", ... }} }} </functioncall> [USER] {message_text}'})
|
248 |
+
|
249 |
+
response = client_gemma.chat_completion(func_caller, max_tokens=200)
|
250 |
+
response = str(response)
|
251 |
+
try:
|
252 |
+
response = response[response.find("{"):response.index("</")]
|
253 |
+
except:
|
254 |
+
response = response[response.find("{"):(response.rfind("}")+1)]
|
255 |
+
response = response.replace("\\n", "")
|
256 |
+
response = response.replace("\\'", "'")
|
257 |
+
response = response.replace('\\"', '"')
|
258 |
+
response = response.replace('\\', '')
|
259 |
+
print(f"\n{response}")
|
260 |
+
|
261 |
+
try:
|
262 |
+
json_data = json.loads(str(response))
|
263 |
+
if json_data["name"] == "web_search":
|
264 |
+
query = json_data["arguments"]["query"]
|
265 |
+
|
266 |
+
gr.Info("Searching Web")
|
267 |
+
yield "Searching Web"
|
268 |
+
web_results = search(query)
|
269 |
+
|
270 |
+
gr.Info("Extracting relevant Info")
|
271 |
+
yield "Extracting Relevant Info"
|
272 |
+
web2 = ' '.join([f"Link: {res['link']}\nText: {res['text']}\n\n" for res in web_results])
|
273 |
+
|
274 |
+
try:
|
275 |
+
message_groq = []
|
276 |
+
message_groq.append({"role":"system", "content": "You are OpenGPT 4o a helpful and powerful assistant made by KingNish. a helpful and very powerful chatbot web assistant made by KingNish. You are provided with WEB results from which you can find informations to answer users query in Structured, Deatailed and Better way, in Human Style. You are also Expert in every field and also learn and try to answer from contexts related to previous question. Try your best to give best response possible to user. You also try to show emotions using Emojis and reply in detail like human, use short forms, structured format, friendly tone and emotions."})
|
277 |
+
for msg in chat_history:
|
278 |
+
message_groq.append({"role": "user", "content": f"{str(msg[0])}"})
|
279 |
+
message_groq.append({"role": "assistant", "content": f"{str(msg[1])}"})
|
280 |
+
message_groq.append({"role": "user", "content": f"[USER] {str(message_text)} , [WEB RESULTS] {str(web2)}"})
|
281 |
+
stream = client_groq.chat.completions.create(model="llama-3.1-8b-instant", messages=message_groq, max_tokens=4096, stream=True)
|
282 |
+
output = ""
|
283 |
+
for chunk in stream:
|
284 |
+
content = chunk.choices[0].delta.content
|
285 |
+
if content:
|
286 |
+
output += chunk.choices[0].delta.content
|
287 |
+
yield output
|
288 |
+
except Exception as e:
|
289 |
+
messages = f"<|im_start|>system\nYou are OpenGPT 4o a helpful and very powerful chatbot web assistant made by KingNish. You are provided with WEB results from which you can find informations to answer users query in Structured, Better and in Human Way. You do not say Unnecesarry things. You are also Expert in every field and also learn and try to answer from contexts related to previous question. Try your best to give best response possible to user. You also try to show emotions using Emojis and reply in details like human, use short forms, friendly tone and emotions.<|im_end|>"
|
290 |
+
for msg in chat_history:
|
291 |
+
messages += f"\n<|im_start|>user\n{str(msg[0])}<|im_end|>"
|
292 |
+
messages += f"\n<|im_start|>assistant\n{str(msg[1])}<|im_end|>"
|
293 |
+
messages+=f"\n<|im_start|>user\n{message_text}<|im_end|>\n<|im_start|>web_result\n{web2}<|im_end|>\n<|im_start|>assistant\n"
|
294 |
+
|
295 |
+
stream = client_mixtral.text_generation(messages, max_new_tokens=4000, do_sample=True, stream=True, details=True, return_full_text=False)
|
296 |
+
output = ""
|
297 |
+
for response in stream:
|
298 |
+
if not response.token.text == "<|im_end|>":
|
299 |
+
output += response.token.text
|
300 |
+
yield output
|
301 |
+
|
302 |
+
elif json_data["name"] == "image_generation":
|
303 |
+
query = json_data["arguments"]["query"]
|
304 |
+
gr.Info("Generating Image, Please wait 10 sec...")
|
305 |
+
yield "Generating Image, Please wait 10 sec..."
|
306 |
+
image = image_gen(f"{str(query)}")
|
307 |
+
yield gr.Image(image[1])
|
308 |
+
|
309 |
+
elif json_data["name"] == "video_generation":
|
310 |
+
query = json_data["arguments"]["query"]
|
311 |
+
gr.Info("Generating Video, Please wait 15 sec...")
|
312 |
+
yield "Generating Video, Please wait 15 sec..."
|
313 |
+
video = video_gen(f"{str(query)}")
|
314 |
+
yield gr.Video(video)
|
315 |
+
|
316 |
+
elif json_data["name"] == "image_qna":
|
317 |
+
inputs = llava(user_prompt, chat_history)
|
318 |
+
streamer = TextIteratorStreamer(processor, skip_prompt=True, **{"skip_special_tokens": True})
|
319 |
+
generation_kwargs = dict(inputs, streamer=streamer, max_new_tokens=1024)
|
320 |
+
|
321 |
+
thread = Thread(target=model.generate, kwargs=generation_kwargs)
|
322 |
+
thread.start()
|
323 |
+
|
324 |
+
buffer = ""
|
325 |
+
for new_text in streamer:
|
326 |
+
buffer += new_text
|
327 |
+
yield buffer
|
328 |
+
|
329 |
+
elif json_data["name"] == "hard_query":
|
330 |
+
try:
|
331 |
+
message_groq = []
|
332 |
+
message_groq.append({"role":"system", "content": "You are OpenGPT 4o a helpful and powerful assistant made by KingNish. You answers users query in detail and structured format and style like human. You are also Expert in every field and also learn and try to answer from contexts related to previous question. You also try to show emotions using Emojis and reply like human, use short forms, structured manner, detailed explaination, friendly tone and emotions."})
|
333 |
+
for msg in chat_history:
|
334 |
+
message_groq.append({"role": "user", "content": f"{str(msg[0])}"})
|
335 |
+
message_groq.append({"role": "assistant", "content": f"{str(msg[1])}"})
|
336 |
+
message_groq.append({"role": "user", "content": f"{str(message_text)}"})
|
337 |
+
stream = client_groq.chat.completions.create(model="llama-3.1-70b-versatile", messages=message_groq, max_tokens=4096, stream=True)
|
338 |
+
output = ""
|
339 |
+
for chunk in stream:
|
340 |
+
content = chunk.choices[0].delta.content
|
341 |
+
if content:
|
342 |
+
output += chunk.choices[0].delta.content
|
343 |
+
yield output
|
344 |
+
except Exception as e:
|
345 |
+
print(e)
|
346 |
+
try:
|
347 |
+
message_groq = []
|
348 |
+
message_groq.append({"role":"system", "content": "You are OpenGPT 4o a helpful and powerful assistant made by KingNish. You answers users query in detail and structured format and style like human. You are also Expert in every field and also learn and try to answer from contexts related to previous question. You also try to show emotions using Emojis and reply like human, use short forms, structured manner, detailed explaination, friendly tone and emotions."})
|
349 |
+
for msg in chat_history:
|
350 |
+
message_groq.append({"role": "user", "content": f"{str(msg[0])}"})
|
351 |
+
message_groq.append({"role": "assistant", "content": f"{str(msg[1])}"})
|
352 |
+
message_groq.append({"role": "user", "content": f"{str(message_text)}"})
|
353 |
+
stream = client_groq.chat.completions.create(model="llama3-70b-8192", messages=message_groq, max_tokens=4096, stream=True)
|
354 |
+
output = ""
|
355 |
+
for chunk in stream:
|
356 |
+
content = chunk.choices[0].delta.content
|
357 |
+
if content:
|
358 |
+
output += chunk.choices[0].delta.content
|
359 |
+
yield output
|
360 |
+
except Exception as e:
|
361 |
+
print(e)
|
362 |
+
message_groq = []
|
363 |
+
message_groq.append({"role":"system", "content": "You are OpenGPT 4o a helpful and powerful assistant made by KingNish. You answers users query in detail and structured format and style like human. You are also Expert in every field and also learn and try to answer from contexts related to previous question. You also try to show emotions using Emojis and reply like human, use short forms, structured manner, detailed explaination, friendly tone and emotions."})
|
364 |
+
for msg in chat_history:
|
365 |
+
message_groq.append({"role": "user", "content": f"{str(msg[0])}"})
|
366 |
+
message_groq.append({"role": "assistant", "content": f"{str(msg[1])}"})
|
367 |
+
message_groq.append({"role": "user", "content": f"{str(message_text)}"})
|
368 |
+
stream = client_groq.chat.completions.create(model="llama3-groq-70b-8192-tool-use-preview", messages=message_groq, max_tokens=4096, stream=True)
|
369 |
+
output = ""
|
370 |
+
for chunk in stream:
|
371 |
+
content = chunk.choices[0].delta.content
|
372 |
+
if content:
|
373 |
+
output += chunk.choices[0].delta.content
|
374 |
+
yield output
|
375 |
+
else:
|
376 |
+
try:
|
377 |
+
message_groq = []
|
378 |
+
message_groq.append({"role":"system", "content": "You are OpenGPT 4o a helpful and powerful assistant made by KingNish. You answers users query in detail and structured format and style like human. You are also Expert in every field and also learn and try to answer from contexts related to previous question. You also try to show emotions using Emojis and reply like human, use short forms, structured manner, detailed explaination, friendly tone and emotions."})
|
379 |
+
for msg in chat_history:
|
380 |
+
message_groq.append({"role": "user", "content": f"{str(msg[0])}"})
|
381 |
+
message_groq.append({"role": "assistant", "content": f"{str(msg[1])}"})
|
382 |
+
message_groq.append({"role": "user", "content": f"{str(message_text)}"})
|
383 |
+
stream = client_groq.chat.completions.create(model="llama3-70b-8192", messages=message_groq, max_tokens=4096, stream=True)
|
384 |
+
output = ""
|
385 |
+
for chunk in stream:
|
386 |
+
content = chunk.choices[0].delta.content
|
387 |
+
if content:
|
388 |
+
output += chunk.choices[0].delta.content
|
389 |
+
yield output
|
390 |
+
except Exception as e:
|
391 |
+
print(e)
|
392 |
+
try:
|
393 |
+
message_groq = []
|
394 |
+
message_groq.append({"role":"system", "content": "You are OpenGPT 4o a helpful and powerful assistant made by KingNish. You answers users query in detail and structured format and style like human. You are also Expert in every field and also learn and try to answer from contexts related to previous question. You also try to show emotions using Emojis and reply like human, use short forms, structured manner, detailed explaination, friendly tone and emotions."})
|
395 |
+
for msg in chat_history:
|
396 |
+
message_groq.append({"role": "user", "content": f"{str(msg[0])}"})
|
397 |
+
message_groq.append({"role": "assistant", "content": f"{str(msg[1])}"})
|
398 |
+
message_groq.append({"role": "user", "content": f"{str(message_text)}"})
|
399 |
+
stream = client_groq.chat.completions.create(model="llama3-8b-8192", messages=message_groq, max_tokens=4096, stream=True)
|
400 |
+
output = ""
|
401 |
+
for chunk in stream:
|
402 |
+
content = chunk.choices[0].delta.content
|
403 |
+
if content:
|
404 |
+
output += chunk.choices[0].delta.content
|
405 |
+
yield output
|
406 |
+
except Exception as e:
|
407 |
+
print(e)
|
408 |
+
messages = f"<|start_header_id|>system\nYou are OpenGPT 4o a helpful and powerful assistant made by KingNish. You answers users query in detail and structured format and style like human. You are also Expert in every field and also learn and try to answer from contexts related to previous question. You also try to show emotions using Emojis and reply like human, use short forms, structured manner, detailed explaination, friendly tone and emotions.<|end_header_id|>"
|
409 |
+
for msg in chat_history:
|
410 |
+
messages += f"\n<|start_header_id|>user\n{str(msg[0])}<|end_header_id|>"
|
411 |
+
messages += f"\n<|start_header_id|>assistant\n{str(msg[1])}<|end_header_id|>"
|
412 |
+
messages+=f"\n<|start_header_id|>user\n{message_text}<|end_header_id|>\n<|start_header_id|>assistant\n"
|
413 |
+
stream = client_llama.text_generation(messages, max_new_tokens=2000, do_sample=True, stream=True, details=True, return_full_text=False)
|
414 |
+
output = ""
|
415 |
+
for response in stream:
|
416 |
+
if not response.token.text == "<|eot_id|>":
|
417 |
+
output += response.token.text
|
418 |
+
yield output
|
419 |
+
except Exception as e:
|
420 |
+
print(e)
|
421 |
+
try:
|
422 |
+
message_groq = []
|
423 |
+
message_groq.append({"role":"system", "content": "You are OpenGPT 4o a helpful and powerful assistant made by KingNish. You answers users query in detail and structured format and style like human. You are also Expert in every field and also learn and try to answer from contexts related to previous question. You also try to show emotions using Emojis and reply like human, use short forms, structured manner, detailed explaination, friendly tone and emotions."})
|
424 |
+
for msg in chat_history:
|
425 |
+
message_groq.append({"role": "user", "content": f"{str(msg[0])}"})
|
426 |
+
message_groq.append({"role": "assistant", "content": f"{str(msg[1])}"})
|
427 |
+
message_groq.append({"role": "user", "content": f"{str(message_text)}"})
|
428 |
+
stream = client_groq.chat.completions.create(model="llama3-8b-8192", messages=message_groq, max_tokens=4096, stream=True)
|
429 |
+
output = ""
|
430 |
+
for chunk in stream:
|
431 |
+
content = chunk.choices[0].delta.content
|
432 |
+
if content:
|
433 |
+
output += chunk.choices[0].delta.content
|
434 |
+
yield output
|
435 |
+
except Exception as e:
|
436 |
+
print(e)
|
437 |
+
messages = f"<|im_start|>system\nYou are OpenGPT 4o a helpful and powerful assistant made by KingNish. You answers users query in detail and structured format and style like human. You are also Expert in every field and also learn and try to answer from contexts related to previous question. You also try to show emotions using Emojis and reply like human, use short forms, structured manner, detailed explaination, friendly tone and emotions.<|im_end|>"
|
438 |
+
for msg in chat_history:
|
439 |
+
messages += f"\n<|im_start|>user\n{str(msg[0])}<|im_end|>"
|
440 |
+
messages += f"\n<|im_start|>assistant\n{str(msg[1])}<|im_end|>"
|
441 |
+
messages+=f"\n<|im_start|>user\n{message_text}<|im_end|>\n<|im_start|>assistant\n"
|
442 |
+
stream = client_mixtral.text_generation(messages, max_new_tokens=4000, do_sample=True, stream=True, details=True, return_full_text=False)
|
443 |
+
output = ""
|
444 |
+
for response in stream:
|
445 |
+
if not response.token.text == "<|im_end|>":
|
446 |
+
output += response.token.text
|
447 |
+
yield output
|
448 |
+
|
449 |
# Create a chatbot interface
|
450 |
chatbot = gr.Chatbot(
|
451 |
label="OpenGPT-4o",
|
452 |
avatar_images=[None, BOT_AVATAR],
|
453 |
show_copy_button=True,
|
454 |
likeable=True,
|
455 |
+
layout="panel",
|
456 |
+
height=400,
|
457 |
)
|
458 |
output = gr.Textbox(label="Prompt")
|